Report Progress Of Task¶
Problem¶
You want to report progress from the task to the client for monitoring purposes.
Solution¶
First, to receive the progress messages sent from the task to the client, subscribe to the job’s
task_progress_updated
event or
the task’s progress_updated
event.
1job.task_progress_updated = job_task_progress_updated # add event handler
Then, on the task, call set_progress()
.
The values passed to set_progress()
will be received on the client’s progress event handling method.
1self.set_progress(40, 4)
1from agiparallel.client import ClusterJobScheduler
2from time import sleep
3
4
5class ProgressTask():
6 def __init__(self):
7 self.name = "progress task"
8 self.result = None
9
10 def execute(self):
11 self.set_progress(0, 1)
12 sleep(1)
13 self.set_progress(20, "B")
14 sleep(1)
15 self.set_progress(40, 4)
16 sleep(1)
17 self.set_progress(60, "D")
18 sleep(1)
19 self.set_progress(80, "E")
20 sleep(1)
21
22
23def job_task_progress_updated(task_id, task_progress_info):
24 print(task_progress_info.progress, task_progress_info.additional_information)
25
26
27def report_progress_of_task():
28 with ClusterJobScheduler("localhost") as scheduler:
29 scheduler.connect()
30 job = scheduler.create_job()
31
32 job.add_task(ProgressTask())
33 job.task_progress_updated = job_task_progress_updated # add event handler
34
35 job.submit()
36 job.wait_until_done()
37
38 # The output should resemble:
39 # 0 1
40 # 20 B
41 # 40 4
42 # 60 D
43 # 80 E
44
45
46if __name__ == "__main__":
47 report_progress_of_task()
Discussion¶
If a job could potentially take a long time to finish, reporting the progress of tasks while the task is running helps to notify the user how long the task could take. You can read more about working with task progress here.
To view the progress through the monitoring applications, open the task monitor (through the coordinator or agent tray application). Right click on the column headings and then click on “Status Description” on the drop down menu.
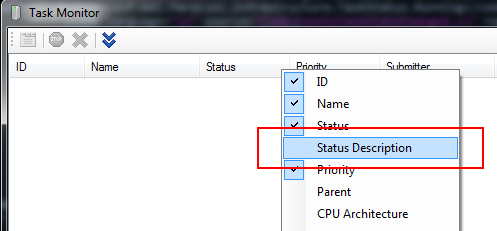
Once the Status Description column is visible, the value of the task progress description can be seen. This is shown in the example above.
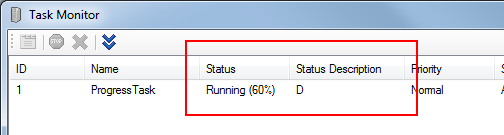