ConicSensor#innerHalfAngle
and outer ConicSensor#outerHalfAngle
cone angles,
minimum ConicSensor#minimumClockAngle
and maximum ConicSensor#maximumClockAngle
clock angles, and a radius
(ConicSensor#radius
). The sensor's principal direction is along the positive z-axis. Clock
angles are angles around the z-axis, rotating x into y. Cone angles are angles from the z-axis towards the xy plane.
The shape also depends on if the sensor intersects the ellipsoid, as shown in examples 3 and 4 below, and what
surfaces are shown using properties such as ConicSensor#showDomeSurfaces
.
Code Example 1 below![]() |
Code Example 2 below![]() |
Code Example 3 below![]() |
A sensor points along the local positive z-axis and is positioned and oriented using
ConicSensor#modelMatrix
.
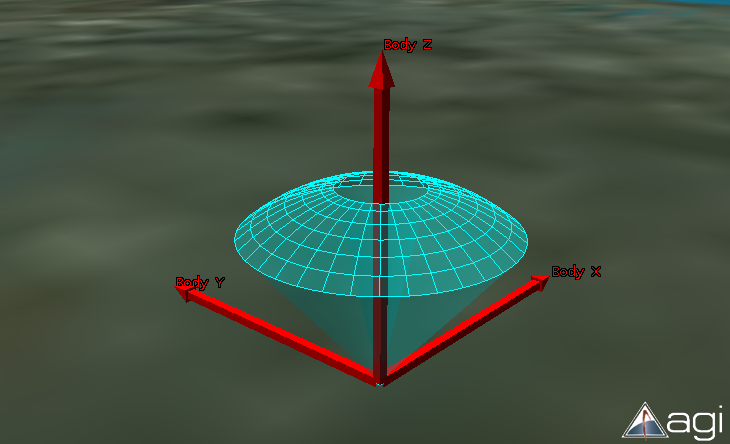
Name | Type | Description | ||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
options |
Object |
optional
Object with the following properties:
|
Examples:
// Example 1. Sensor on the ground pointing straight up
var sensor = scene.primitives.add(new Cesium.ConicSensor({
modelMatrix : Cesium.Transforms.eastNorthUpToFixedFrame(Cesium.Cartesian3.fromDegrees(-123.0744619, 44.0503706)),
radius : 1000000.0,
innerHalfAngle : Cesium.Math.toRadians(5.0),
outerHalfAngle : Cesium.Math.toRadians(85.0)
}));
// Example 2. Sensor pointing straight down with its dome intersecting the ellipsoid
var sensor = scene.primitives.add(new Cesium.ConicSensor({
modelMatrix : Cesium.Transforms.northEastDownToFixedFrame(Cesium.Cartesian3.fromDegrees(-123.0744619, 44.0503706, 900000.0)),
radius : 1000000.0,
innerHalfAngle : Cesium.Math.toRadians(0.0),
outerHalfAngle : Cesium.Math.toRadians(40.0),
minimumClockAngle : Cesium.Math.toRadians(-30.0),
maximumClockAngle : Cesium.Math.toRadians(30.0),
lateralSurfaceMaterial : Cesium.Material.fromType(Cesium.Material.StripeType),
intersectionColor : Cesium.Color.YELLOW
}));
// Example 3. Sensor with custom materials for each surface. Switch to 2D to see the ellipsoid surface material.
var sensor = scene.primitives.add(new Cesium.ConicSensor({
modelMatrix : Cesium.Transforms.northEastDownToFixedFrame(Cesium.Cartesian3.fromDegrees(-123.0744619, 44.0503706, 9000000.0)),
radius : 20000000.0,
innerHalfAngle : Cesium.Math.toRadians(15.0),
outerHalfAngle : Cesium.Math.toRadians(40.0),
minimumClockAngle : Cesium.Math.toRadians(-60.0),
maximumClockAngle : Cesium.Math.toRadians(60.0),
lateralSurfaceMaterial : Cesium.Material.fromType(Cesium.Material.ColorType, { color : new Cesium.Color(1.0, 0.0, 0.0, 0.5) }),
ellipsoidHorizonSurfaceMaterial : Cesium.Material.fromType(Cesium.Material.ColorType, { color : new Cesium.Color(0.0, 1.0, 0.0, 0.5) }),
ellipsoidSurfaceMaterial : Cesium.Material.fromType(Cesium.Material.ColorType, { color : new Cesium.Color(0.0, 0.0, 1.0, 0.5) }),
domeSurfaceMaterial : Cesium.Material.fromType(Cesium.Material.ColorType, { color : new Cesium.Color(1.0, 1.0, 1.0, 0.5) })
}));
See:
Members
When true
, draws the bounding sphere for each DrawCommand
in the sensor.
-
Default Value:
false
When true
, draws the points where the sensor boundary crosses off of and onto the ellipsoid.
-
Default Value:
false
When true
, draws the proxy geometry used for shading the dome and ellipsoid horizon surfaces of the sensor boundary.
-
Default Value:
false
When true
, draws a bounding volume around the light source for the shadow map used for environment intersections.
Also, the contents of the shadow map are drawn to a viewport quad.
-
Default Value:
false
domeSurfaceMaterial : Material
Material
objects or a custom material, scripted with
Fabric.
When undefined
, CustomPatternSensor#lateralSurfaceMaterial
is used.
-
Default Value:
undefined
Examples:
// Change the color of the dome surface material to yellow
sensor.domeSurfaceMaterial.uniforms.color = Cesium.Color.YELLOW;
// Change material to horizontal stripes
sensor.domeSurfaceMaterial = Material.fromType(Material.StripeType);
See:
readonly ellipsoid : Ellipsoid
-
Default Value:
Ellipsoid.WGS84
ellipsoidHorizonSurfaceMaterial : Material
Material
objects or a custom material, scripted with
Fabric.
When undefined
, CustomPatternSensor#lateralSurfaceMaterial
is used.
-
Default Value:
undefined
Examples:
// Change the color of the ellipsoid horizon surface material to yellow
sensor.ellipsoidHorizonSurfaceMaterial.uniforms.color = Cesium.Color.YELLOW;
// Change material to horizontal stripes
sensor.ellipsoidHorizonSurfaceMaterial = Cesium.Material.fromType(Cesium.Material.StripeType);
See:
ellipsoidSurfaceMaterial : Material
Material
objects or a custom material, scripted with
Fabric.
When undefined
, CustomPatternSensor#lateralSurfaceMaterial
is used.
-
Default Value:
undefined
Examples:
// Change the color of the ellipsoid surface material to yellow
sensor.ellipsoidSurfaceMaterial.uniforms.color = new Cesium.Color.YELLOW;
// Change material to horizontal stripes
sensor.ellipsoidSurfaceMaterial = Material.fromType(Material.StripeType);
See:
true
, a sensor intersecting the environment, e.g. terrain or models, will discard the portion of the sensor that is occluded.
-
Default Value:
false
environmentIntersectionColor : Color
-
Default Value:
Color.WHITE
-
Default Value:
5.0
environmentOcclusionMaterial : Material
-
Default Value:
Material.ColorType
-
Default Value:
undefined
See:
-
Default Value:
0.0
intersectionColor : Color
-
Default Value:
Color.WHITE
See:
-
Default Value:
5.0
See:
lateralSurfaceMaterial : Material
Material
objects or a custom material, scripted with
Fabric.
-
Default Value:
Material.ColorType
Examples:
// Change the color of the default material to yellow
sensor.lateralSurfaceMaterial.uniforms.color = Cesium.Color.YELLOW;
// Change material to horizontal stripes
sensor.lateralSurfaceMaterial = Cesium.Material.fromType(Cesium.Material.StripeType);
See:
-
Default Value:
CesiumMath.TWO_PI
-
Default Value:
0.0
modelMatrix : Matrix4
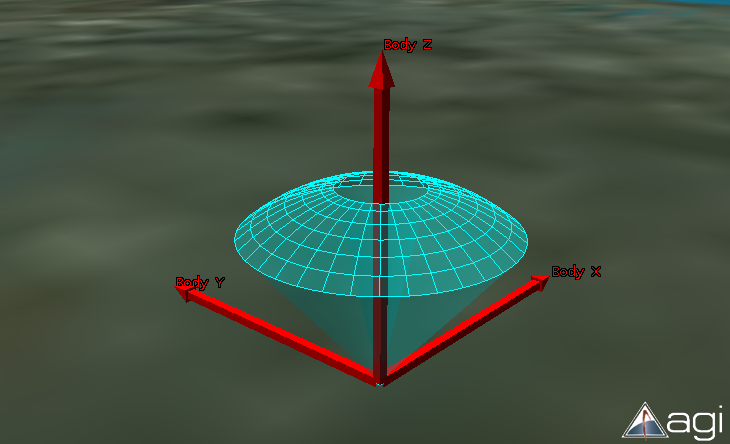
-
Default Value:
Matrix4.IDENTITY
Example:
// The sensor's origin is located on the surface at -75.59777 degrees longitude and 40.03883 degrees latitude.
// The sensor opens upward, along the surface normal.
var center = Cesium.Cartesian3.fromDegrees(-75.59777, 40.03883);
sensor.modelMatrix = Cesium.Transforms.eastNorthUpToFixedFrame(center);
-
Default Value:
CesiumMath.PI_OVER_TWO
portionToDisplay : SensorVolumePortionToDisplay
COMPLETE ![]() |
BELOW_ELLIPSOID_HORIZON ![]() |
ABOVE_ELLIPSOID_HORIZON ![]() |
-
Default Value:
SensorVolumePortionToDisplay.COMPLETE
-
Default Value:
Number.POSITIVE_INFINITY
true
, the sensor is shown.
-
Default Value:
true
true
, the sensor dome surfaces are shown.
These surfaces are only shown in 3D (see Scene#mode
).
Full sensor![]() |
Dome only![]() |
-
Default Value:
true
See:
true
, the ellipsoid horizon surfaces, i.e., the sides formed from occlusion due to the ellipsoid hoirzon, are shown.
These surfaces are only shown in 3D (see Scene#mode
).
Full sensor![]() |
Ellipsoid horizon surfaces only![]() |
-
Default Value:
true
See:
true
, the ellipsoid/sensor intersection surfaces are shown.
These surfaces are only shown in 2D and Columbus View (see Scene#mode
).
-
Default Value:
true
See:
true
, a line is shown where the sensor intersections the environment, e.g. terrain or models.
-
Default Value:
false
true
, the portion of the sensor occluded by the environment will be drawn with ConicSensor#environmentOcclusionMaterial
.
ConicSensor#environmentConstraint
must also be true
.
-
Default Value:
false
true
, a polyline is shown where the sensor intersections the ellipsoid.
showIntersection : false ![]() |
showIntersection : true ![]() |
-
Default Value:
true
See:
true
, the sensor's lateral surfaces, i.e., the outer sides of the sensor, are shown.
These surfaces are only shown in 3D (see Scene#mode
).
Full sensor![]() |
Lateral surfaces only![]() |
-
Default Value:
true
See:
When true
, a sensor intersecting the ellipsoid is drawn through the ellipsoid and potentially out
to the other side.
showThroughEllipsoid : false ![]() |
showThroughEllipsoid : true ![]() |
-
Default Value:
false
Methods
Once an object is destroyed, it should not be used; calling any function other than
isDestroyed
will result in a DeveloperError
exception. Therefore,
assign the return value (undefined
) to the object as done in the example.
Returns:
Throws:
-
DeveloperError : This object was destroyed, i.e., destroy() was called.
Example:
sensor = sensor && sensor.destroy();
If this object was destroyed, it should not be used; calling any function other than
isDestroyed
will result in a DeveloperError
exception.
Returns:
true
if this object was destroyed; otherwise, false
.
Viewer
or CesiumWidget
render the scene to
get the draw commands needed to render this primitive.
Do not call this function directly. This is documented just to list the exceptions that may be propagated when the scene is rendered:
Throws:
-
DeveloperError : this.radius must be greater than or equal to zero.
-
DeveloperError : this.lateralSurfaceMaterial must be defined.
-
DeveloperError : this.innerHalfAngle must be between zero and pi.
-
DeveloperError : this.outerHalfAngle must be between zero and pi.
-
DeveloperError : this.innerHalfAngle must be less than this.outerHalfAngle.
-
DeveloperError : this.minimumClockAngle must be between negative two pi and positive two pi.
-
DeveloperError : this.maximumClockAngle must be between negative two pi and positive two pi.
-
DeveloperError : this.minimumClockAngle must be less than this.maximumClockAngle.